Tomcat Architecture
Three major components of Java Web
When the Tomcat receive a request, the request will pass Listener -> Filter -> Servlet orderly.
Servlet
Servlet is a program that is used to handle some requests and responses that have been encapsulated.
We can write a demo to have a better understand.
1 | package com.example.servletdemo; |
When we visit the route /servlet
, this program can deal with our request.
The init
method will only be called at the first time we visit the route.
The service
method will be called when we send a request to this servlet. In class HttpServlet
, developer has helped us to transform the ServletRequest
and ServletRequest
into HttpServletRequest
and HttpServletResponse
. Besides, HttpSevlet
writes different methods for different http request methods(e.g. GET and doGET)
The destroy
method will be called when the servlet has been destroyed(e.g. the Server be closed)
Filter
Filter is a program for intercepting and inspecting ServletRequest and ServletResponse.
1 | package com.example.servletdemo; |
We can find that it has a similar structure to the servlet.
Listener
Listener is used to monitor the creation, destruction, modification of the Application, Session, and Request.
Apache and Tomcat
Apache is used to deal with the static resource and forward the request of dynamic resource.
Tomcat is a servlet container, like an extension of the Apache, but it can run independent of Apache.
There is a vivid metaphor that the Apache is like a truck, it can carry some stuff(e.g. html) directly, but it can’t carry the water(e.g. servlet) directly. The water should be stored in a container, and.the container is Tomcat.
Tomcat Architecture
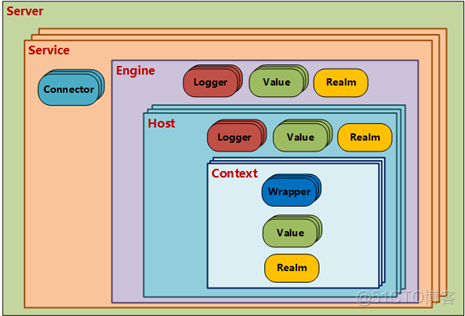
Generally, there are four components in Tomcat, that is, Server, Service, Connector, Container.
Tow major components are: Connector and Container.
Server
It represents the whole Tomcat server, and it should maintain the lifecycle of all services it contains, including how to initialize and end services
Service
A Service is a group of one or more Connectors that share a single Container to process their incoming requests. This arrangement allows, for example, a non-SSL and SSL connector to share the same population of web apps.
The standard implementation class for the Service interface in Tomcat is StandardService, which not only implements the Service interface but also the Lifecycle interface, allowing it to control the lifecycle of its underlying components
Connector
It is used to receive TCP connection requests from the browser, create a Request and Response object for exchanging data with the user, and then generate a thread to process the request and pass the generated Request and Response object to the thread(container) processing the request.
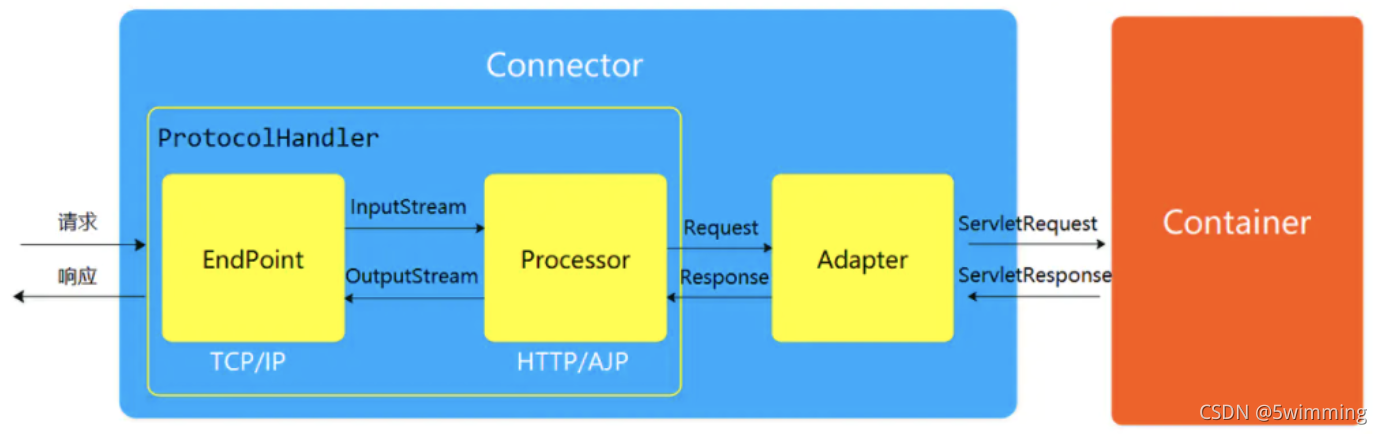
Container
Container(also called Catalina) is used to handle servlet connection requests sent by the connector. It has four sub container, that is, Engine, Host, Context, Wrapper.
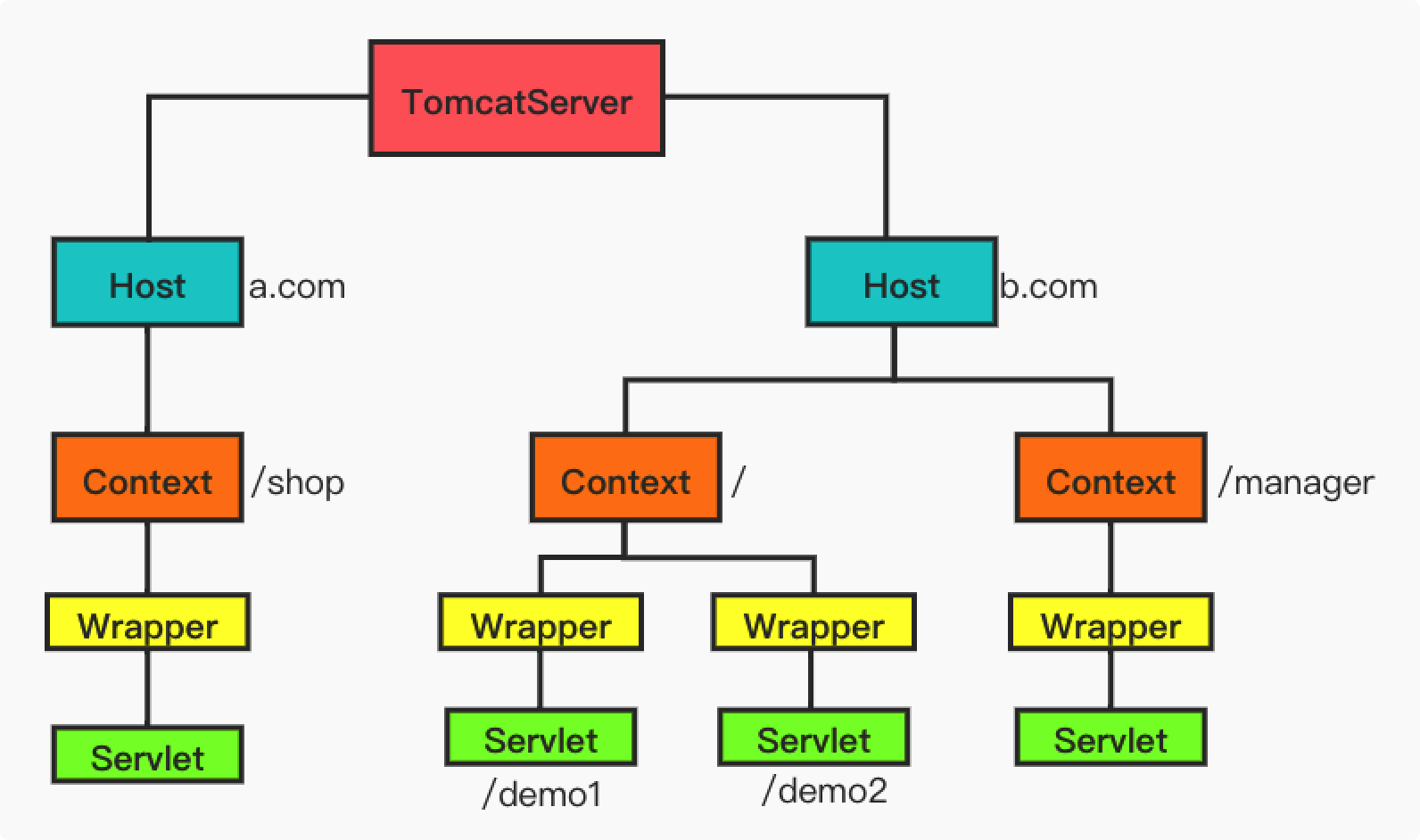
Engine
An Engine is a Container that represents the entire Catalina servlet engine.
Host
A Host is a Container that represents a virtual host in the Catalina servlet engine.
Context
A Context is a Container that represents a servlet context, and therefore an individual web application, in the Catalina servlet engine.
Wrapper
A Wrapper corresponds to a Servlet and is responsible for managing the Servlet, including Servlet loading, initialization, execution, and resource recovery.